I won't write a lot of words in here...
Environment: SP Online, Publishing Portal
Problem: jQuery.off(), jQuery.unbind(), element.removeEventHandler didn't work so we need to find a way to remove event handlers.
Solution:
SharePoint uses Sys.UI.DomEvent.addHandler (alias $addHandler) and Sys.UI.DomEvent.addHandlers (alias $addHandlers) to add DOM events handlers.
If you want to delete absolutely all handler for all events everything is simple - just call Sys.UI.DomEvent.clearHandlers and put your DOM element as a parameter.
But what if you want to delete handlers of particular event?..
In that case you can use Sys.UI.DomEvent.removeHandler (alias $removeHandler) to remove added handlers... But the thing is that you need to know the handlers' functions to use this method.
After some investigation I've found that added handlers are stored in _events attribute in DOM element:
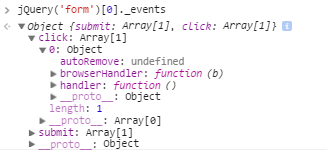
And here is an example of code to use to remove all 'click' handlers from 'form' element:
var $form = jQuery('form');
if ($form.length) {
var formEvents = $form[0]._events;
if (formEvents && formEvents.click) {
jQuery.each(formEvents.click, function (clickIndex, clickHandler) {
if (clickHandler.handler)
$removeHandler($form[0], 'click', clickHandler.handler);
});
}
}
Have Fun!
Comments