This is the first post of a series where I'm planning to describe how to use Vue.js in SharePoint Framework apps.
In this post I want to provide a small introduction to Vue.js as well as describe the next parts and what to expect.
List of posts:
- Whats and Whys (this post)
- Default SPFx web part using Vue.js
- Yeoman Generator with Vue Support
- Web Part Property Pane Control
- Use React Components inside Vue.js Solution
Why did I decide to write these posts?
There are several reasons why this topic may be interesting for the community.First of all, Vue.js is very popular. It means that many front-end developers prefer to use it in their projects. And if they need to implement SPFx solution they'll use Vue.js there as well.
The second reason is that I've heard that SharePoint community is also interested in Vue.js as an additional instrument in the toolset.
And the last one is my own interest in Vue.js. And it's much easier to learn something if you have some aims or commitments.
Why multiple posts instead of one or two?
As Vue.js is not included by SharePoint Dev team as one of OOTB templates for the projects, there probably will be a lot of things to do to make Vue.js work with SPFx, React, and Office UI Fabric controls.Moreover, these posts should be some kind of detailed guide on how to create SPFx project using SPFx. And it wouldn't have happened with the single post.
What is Vue.js
Vue (pronounced /vjuː/, like view) is a progressive framework for building user interfaces. Unlike other monolithic frameworks, Vue is designed from the ground up to be incrementally adoptable. The core library is focused on the view layer only, and is easy to pick up and integrate with other libraries or existing projects. On the other hand, Vue is also perfectly capable of powering sophisticated Single-Page Applications when used in combination with modern tooling and supporting libraries.To learn Vue.js in details please visit the official web site of the project: https://vuejs.org/
How to start: Vue.js, TypeScript and Node.js
TypeScript is a default language of the SharePoint Framework. And Node.js is basically the core engine for modern web application.As the first 2 posts we're talking about Vue.js itself, without SharePoint Framework. But it's better to use the same toolchain (or its part) for the start to be familiar with what's going on under the hood of SPFx.
The best way to start is to use step-by-step guidance for TypeScript-Vue-Starter repo. It describes how to init the project, install needed modules, configure settings, etc. I will briefly describe the steps here as well as there are some differences in configurations between the TypeScript-Vue-Starter and SPFx projects.
1. Init the Project
First, let's create a basic folder structure for the project.In TypeScript projects source (.ts) files are usually stored in src folder and compiled (.js) files are stored in dist folder.
Also, let's create components subfolder in src folder to contain the code for our components.
The initial folder structure will look like the one on the image below:
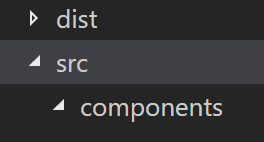
The next step is to initialize the project using npm. It is done with the next command:
npm init
2. Install Dependencies
We need to install TypeScript, Webpack and Vue to our project.It can be done with the next command:
npm i --save-dev typescript webpack webpack-cli
npm i --save vue
But in real projects Webpack might be helpful... And it is used in SharePoint Framework, that's why I decided to use it in simple sample as well.
3. Add TypeScript Configuration
As we're going to use Vuejs with SharePoint Framework, let's use the same TypeScript settings as used in SPFx projects. Create a tsconfig.json file in the root of the project with the next content:{
"compilerOptions": {
"outDir": "./built", // this property is absent in SPFx projects
"target": "es5",
"forceConsistentCasingInFileNames": true,
"module": "commonjs",
"jsx": "react",
"declaration": true,
"sourceMap": true,
//"experimentalDecorators": true,
"skipLibCheck": true,
//"typeRoots": [
// "./node_modules/@types",
// "./node_modules/@microsoft"
//],
"types": [
"es6-promise",
"webpack-env"
],
"lib": [
"es5",
"dom",
"es2015.collection"
],
"include": [
"./src/**/*"
]
}
}
4. Configure Webpack
Now we need to add webpack.config.js file to bundle the app. As I mentioned above, Webpack is optional for this project. So, you can try to create your one without it at all.var path = require('path')
var webpack = require('webpack')
module.exports = {
entry: './src/index.ts',
output: {
path: path.resolve(__dirname, './dist'),
publicPath: '/dist/',
filename: 'build.js'
},
module: {
rules: [
{
test: /\.(png|jpg|gif|svg)$/,
loader: 'file-loader',
options: {
name: '[name].[ext]?[hash]'
}
}
]
},
resolve: {
extensions: ['.ts', '.js', '.vue', '.json'],
alias: {
'vue$': 'vue/dist/vue.esm.js'
}
},
devServer: {
historyApiFallback: true,
noInfo: true
},
performance: {
hints: false
},
devtool: '#eval-source-map'
}
if (process.env.NODE_ENV === 'production') {
module.exports.devtool = '#source-map'
// http://vue-loader.vuejs.org/en/workflow/production.html
module.exports.plugins = (module.exports.plugins || []).concat([
new webpack.DefinePlugin({
'process.env': {
NODE_ENV: '"production"'
}
}),
new webpack.optimize.UglifyJsPlugin({
sourceMap: true,
compress: {
warnings: false
}
}),
new webpack.LoaderOptionsPlugin({
minimize: true
})
])
}
5. Add Build script in Node config
Node allows to include "scripts" into package.json to list a set of actions that can be run from command prompt using node engine.Our aim is to add "build" script that will run Webpack to bundle the project.
To do that add
"build": "webpack"
It should look like that:
"scripts": {
"build": "webpack",
"test": "test"
}
npm run build
6. Basic Project
Let's create a simple Vue component using TypeScript.First, create index.ts file in src folder and add the next content:
import Vue from "vue";
let v = new Vue({
el: "#app",
template: `
<div>
<div>Hello {{name}}!</div>
Name: <input v-model="name" type="text">
</div>`,
data: {
name: "World"
}
});
Now let's create index.html in the root of the folder to test our Vue component:
<!doctype html>
<html>
<head></head>
<body>
<div id="app"></div>
</body>
<script src="./dist/build.js"></script>
</html>
The result should be similar to the one on the image below:
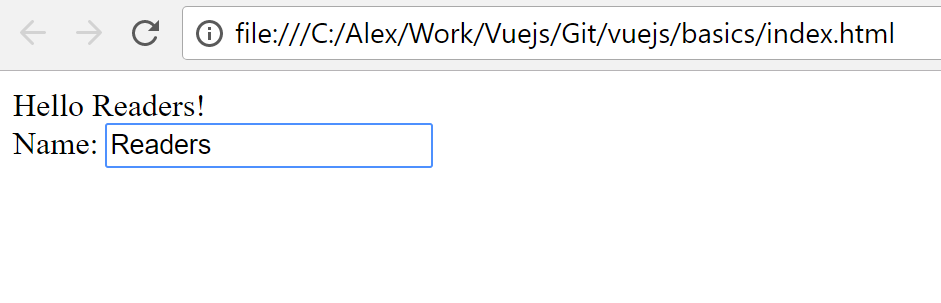
Congrats! Now we have our first project implemented using Vue and TypeScript.
Conclusion
During this post I described what's Vue.js and how it can be used with TypeScript.Now we're closer to adding Vue to the SharePoint Framework solutions.
The code for this example is available here
In the next post I will show how to implement basic web part (the one that is included in the SPFx template) using Vue.
That's it for now!
Have fun!
Comments